AlertDialog(アラートダイアログ)を使った簡易的なギャラリー作成です。
「できる限りプログラムせずに作成する」を目指した内容です。
プロジェクト名
プロジェクト、カンパニードメイン、パッケージネームを同じにするとコピペエラーが減ります。
Application name MyGallery
Company Domain test.com
Package name com.test.mygallery
画像素材
* 注意 *
・画像をご自身で要するにはファイル名やファイルサイズ、拡張子に注意する必要があります。
・ファイル名は半角小文字の英数(a~z 0~9)とアンダーバー( _ )のみで、最初の1文字目は半角英字( a ~ z)のみです。
・慣れてない方はサンプル画像の使用をオススメします。
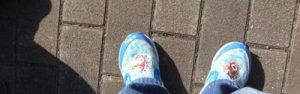
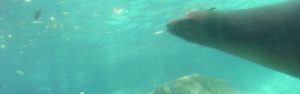
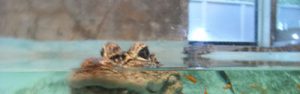
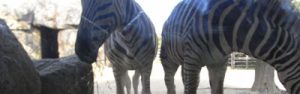
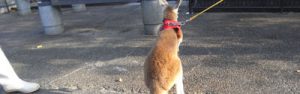
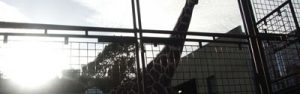
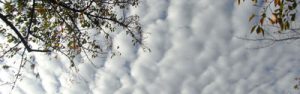
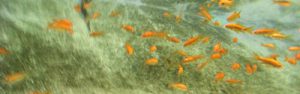
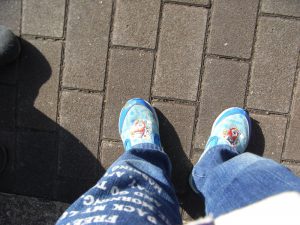
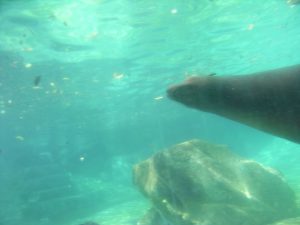
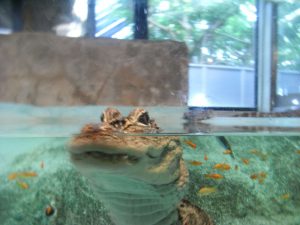
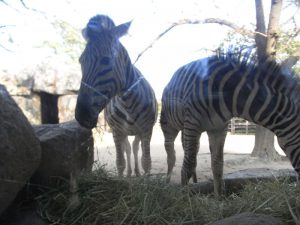
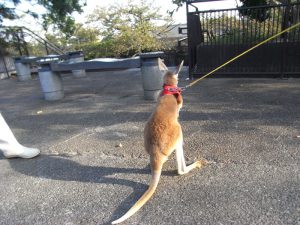
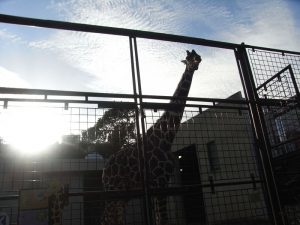
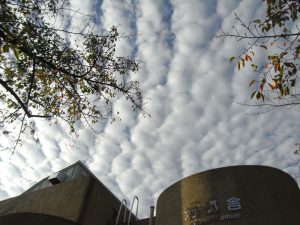
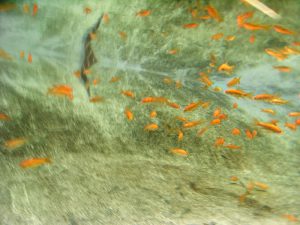
activity_main.xml (完成)
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/activity_main" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.test.mygallery.MainActivity"> <ScrollView android:layout_width="match_parent" android:layout_height="match_parent"> <LinearLayout android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent"> <ImageView android:onClick="img00" android:scaleType="centerCrop" android:src="@drawable/s00" android:layout_width="match_parent" android:layout_height="wrap_content" /> <ImageView android:onClick="img01" android:scaleType="centerCrop" android:src="@drawable/s01" android:layout_width="match_parent" android:layout_height="wrap_content" /> <ImageView android:onClick="img02" android:scaleType="centerCrop" android:src="@drawable/s02" android:layout_width="match_parent" android:layout_height="wrap_content" /> <ImageView android:onClick="img03" android:scaleType="centerCrop" android:src="@drawable/s03" android:layout_width="match_parent" android:layout_height="wrap_content" /> <ImageView android:onClick="img04" android:scaleType="centerCrop" android:src="@drawable/s04" android:layout_width="match_parent" android:layout_height="wrap_content" /> <ImageView android:onClick="img05" android:scaleType="centerCrop" android:src="@drawable/s05" android:layout_width="match_parent" android:layout_height="wrap_content" /> <ImageView android:onClick="img06" android:scaleType="centerCrop" android:src="@drawable/s06" android:layout_width="match_parent" android:layout_height="wrap_content" /> <ImageView android:onClick="img07" android:scaleType="centerCrop" android:src="@drawable/s07" android:layout_width="match_parent" android:layout_height="wrap_content" /> </LinearLayout> </ScrollView> </RelativeLayout>
MainActivity.java(完成)
package com.test.mygallery; import android.os.Bundle; import android.support.v7.app.AlertDialog; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.widget.ImageView; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } public void img00(View v){ ImageView iv = new ImageView(this); iv.setImageResource(R.drawable.img00); iv.setAdjustViewBounds(true); new AlertDialog.Builder(this) .setView(iv) .show(); } public void img01(View v){ ImageView iv = new ImageView(this); iv.setImageResource(R.drawable.img01); iv.setAdjustViewBounds(true); new AlertDialog.Builder(this) .setView(iv) .show(); } public void img02(View v){ ImageView iv = new ImageView(this); iv.setImageResource(R.drawable.img02); iv.setAdjustViewBounds(true); new AlertDialog.Builder(this) .setView(iv) .show(); } public void img03(View v){ ImageView iv = new ImageView(this); iv.setImageResource(R.drawable.img03); iv.setAdjustViewBounds(true); new AlertDialog.Builder(this) .setView(iv) .show(); } public void img04(View v){ ImageView iv = new ImageView(this); iv.setImageResource(R.drawable.img04); iv.setAdjustViewBounds(true); new AlertDialog.Builder(this) .setView(iv) .show(); } public void img05(View v){ ImageView iv = new ImageView(this); iv.setImageResource(R.drawable.img05); iv.setAdjustViewBounds(true); new AlertDialog.Builder(this) .setView(iv) .show(); } public void img06(View v){ ImageView iv = new ImageView(this); iv.setImageResource(R.drawable.img06); iv.setAdjustViewBounds(true); new AlertDialog.Builder(this) .setView(iv) .show(); } public void img07(View v){ ImageView iv = new ImageView(this); iv.setImageResource(R.drawable.img07); iv.setAdjustViewBounds(true); new AlertDialog.Builder(this) .setView(iv) .show(); } }