一覧から選んで動画を再生します。
今回は「画面の切り替え」を行います。
ActivityとIntentを理解していると簡単な動画ですが、初心者の方はほぼ理解不可能な動画です。
「ご自身のPCで再現」「ActivityとIntentの予習」として御覧ください。
難易度:★★★★☆
初心者 :ご自身のPCで再現できるが、詳細は意味不明、アレンジも厳しい。
中級者 :再現と「なんとなく」理解可能。アレンジは厳しい。
経験者 :ActivityとIntentを理解してれば、ソースを見るだけで理解可能。
画面構成
使用素材
学習に使用する素材です。
ご自身で用意する場合はファイル名や拡張し、ファイルサイズにご注意ください。
初心者の方はサンプルを使用することを強くおすすめします。
画像素材
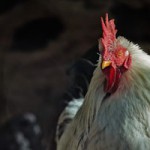
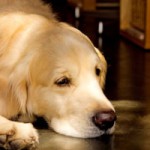
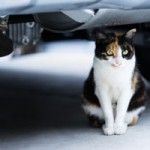
※ 写真素材は【 写真素材ぱくたそ 】様からお借りしています。
動画素材
* 注意点:動画の形式によって再生できないものがありました。
プロジェクト名
プロジェクト、カンパニードメイン、パッケージネームを同じにするとコピペエラーが減ります。
Application name MyVideo
Company Domain test.com
Package name com.test.myvideo
ファイル構成
以下のファイル構成になります。
フォルダ名やファイル名を変更するとエラーになります。
activity_main.xml(完成)
一覧画面を完成させます。
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <ImageView android:src="@drawable/iv0" android:onClick="iv0" android:layout_weight="1" android:scaleType="centerCrop" android:layout_width="match_parent" android:layout_height="match_parent" /> <ImageView android:src="@drawable/iv1" android:onClick="iv1" android:layout_weight="1" android:scaleType="centerCrop" android:layout_width="match_parent" android:layout_height="match_parent" /> <ImageView android:src="@drawable/iv2" android:onClick="iv2" android:layout_weight="1" android:scaleType="centerCrop" android:layout_width="match_parent" android:layout_height="match_parent" /> </LinearLayout>
MainActivity.java(完成)
一覧画面のプログラムを作成します。
package com.test.myvideo; import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } // ボタンクリック public void iv0(View v) { // 画面移行の準備 Intent intent = new Intent(this, Main2Activity.class); // 移行後に再生する動画を指定 intent.putExtra("mp4", R.raw.video0); // 画面移行スタート startActivity(intent); } public void iv1(View v) { Intent intent = new Intent(this, Main2Activity.class); intent.putExtra("mp4", R.raw.video1); startActivity(intent); } public void iv2(View v) { Intent intent = new Intent(this, Main2Activity.class); intent.putExtra("mp4", R.raw.video2); startActivity(intent); } }
activity_main2.xml(完成)
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <VideoView android:id="@+id/v" android:layout_width="match_parent" android:layout_height="match_parent" /> </RelativeLayout>
Main2Activity.java(完成)
package com.test.myvideo; import android.content.Intent; import android.net.Uri; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.widget.MediaController; import android.widget.VideoView; public class Main2Activity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main2); // 元の画面で指定した動画を取得 Intent intent = getIntent(); int mp4 = intent.getIntExtra("mp4",R.raw.video1); // ID取得 VideoView v = (VideoView)findViewById(R.id.v); // 動画の指定 v.setVideoURI(Uri.parse("android.resource://" + this.getPackageName() + "/" + mp4)); // 再生スタート v.start(); // コントローラNO(動画をタップするとメニュー表示) v.setMediaController(new MediaController(this)); } }
AndroidManifest.xml(完成)
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.test.myvideo" > <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:supportsRtl="true" android:theme="@style/AppTheme" > <activity android:name=".MainActivity" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <activity android:name=".Main2Activity" > </activity> </application> </manifest>